p-map alternatives and similar modules
Based on the "Promises" category.
Alternatively, view p-map alternatives based on common mentions on social networks and blogs.
SurveyJS - Open-Source JSON Form Builder to Create Dynamic Forms Right in Your App
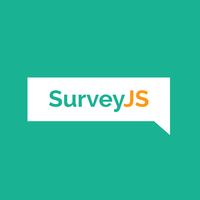
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of p-map or a related project?
Popular Comparisons
README
p-map
Map over promises concurrently
Useful when you need to run promise-returning & async functions multiple times with different inputs concurrently.
This is different from Promise.all()
in that you can control the concurrency and also decide whether or not to stop iterating when there's an error.
Install
$ npm install p-map
Usage
import pMap from 'p-map';
import got from 'got';
const sites = [
getWebsiteFromUsername('sindresorhus'), //=> Promise
'https://avajs.dev',
'https://github.com'
];
const mapper = async site => {
const {requestUrl} = await got.head(site);
return requestUrl;
};
const result = await pMap(sites, mapper, {concurrency: 2});
console.log(result);
//=> ['https://sindresorhus.com/', 'https://avajs.dev/', 'https://github.com/']
API
pMap(input, mapper, options?)
Returns a Promise
that is fulfilled when all promises in input
and ones returned from mapper
are fulfilled, or rejects if any of the promises reject. The fulfilled value is an Array
of the fulfilled values returned from mapper
in input
order.
input
Type: Iterable<Promise | unknown>
Iterated over concurrently in the mapper
function.
mapper(element, index)
Type: Function
Expected to return a Promise
or value.
options
Type: object
concurrency
Type: number
(Integer)\
Default: Infinity
\
Minimum: 1
Number of concurrently pending promises returned by mapper
.
stopOnError
Type: boolean
\
Default: true
When set to false
, instead of stopping when a promise rejects, it will wait for all the promises to settle and then reject with an aggregated error containing all the errors from the rejected promises.
pMapSkip
Return this value from a mapper
function to skip including the value in the returned array.
import pMap, {pMapSkip} from 'p-map';
import got from 'got';
const sites = [
getWebsiteFromUsername('sindresorhus'), //=> Promise
'https://avajs.dev',
'https://example.invalid',
'https://github.com'
];
const mapper = async site => {
try {
const {requestUrl} = await got.head(site);
return requestUrl;
} catch {
return pMapSkip;
}
};
const result = await pMap(sites, mapper, {concurrency: 2});
console.log(result);
//=> ['https://sindresorhus.com/', 'https://avajs.dev/', 'https://github.com/']
p-map for enterprise
Available as part of the Tidelift Subscription.
The maintainers of p-map and thousands of other packages are working with Tidelift to deliver commercial support and maintenance for the open source dependencies you use to build your applications. Save time, reduce risk, and improve code health, while paying the maintainers of the exact dependencies you use. Learn more.
Related
- p-all - Run promise-returning & async functions concurrently with optional limited concurrency
- p-filter - Filter promises concurrently
- p-times - Run promise-returning & async functions a specific number of times concurrently
- p-props - Like
Promise.all()
but forMap
andObject
- p-map-series - Map over promises serially
- p-queue - Promise queue with concurrency control
- Moreā¦