cheerio alternatives and similar modules
Based on the "Miscellaneous" category.
Alternatively, view cheerio alternatives based on common mentions on social networks and blogs.
-
mem
Memoize functions - an optimization technique used to speed up consecutive function calls by caching the result of calls with identical input -
basic-ftp
FTP client for Node.js, supports FTPS over TLS, passive mode over IPv6, async/await, and Typescript. -
schemapack
Create a schema object to encode/decode your JSON in to a compact byte buffer with no overhead. -
nar
node.js application archive - create self-contained binary like executable applications that are ready to ship and run -
Faster than fast, smaller than micro ... nano-memoizer.
Faster than fast, smaller than micro ... a nano speed and size (780 Brotili bytes) memoize for single and multiple argument functions.
SurveyJS - Open-Source JSON Form Builder to Create Dynamic Forms Right in Your App
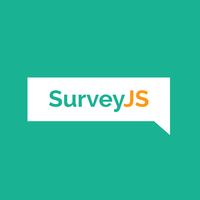
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of cheerio or a related project?
README
cheerio
Fast, flexible & lean implementation of core jQuery designed specifically for the server.
const cheerio = require('cheerio');
const $ = cheerio.load('<h2 class="title">Hello world</h2>');
$('h2.title').text('Hello there!');
$('h2').addClass('welcome');
$.html();
//=> <html><head></head><body><h2 class="title welcome">Hello there!</h2></body></html>
Note
We are currently working on the 1.0.0 release of cheerio on the main
branch. The source code for the last published version, 0.22.0
, can be found here.
Installation
npm install cheerio
Features
❤ Familiar syntax: Cheerio implements a subset of core jQuery. Cheerio removes all the DOM inconsistencies and browser cruft from the jQuery library, revealing its truly gorgeous API.
ϟ Blazingly fast: Cheerio works with a very simple, consistent DOM model. As a result parsing, manipulating, and rendering are incredibly efficient.
❁ Incredibly flexible: Cheerio wraps around parse5 parser and can optionally use @FB55's forgiving htmlparser2. Cheerio can parse nearly any HTML or XML document.
Cheerio is not a web browser
Cheerio parses markup and provides an API for traversing/manipulating the resulting data structure. It does not interpret the result as a web browser does. Specifically, it does not produce a visual rendering, apply CSS, load external resources, or execute JavaScript which is common for a SPA (single page application). This makes Cheerio much, much faster than other solutions. If your use case requires any of this functionality, you should consider browser automation software like Puppeteer and Playwright or DOM emulation projects like JSDom.
API
Markup example we'll be using:
<ul id="fruits">
<li class="apple">Apple</li>
<li class="orange">Orange</li>
<li class="pear">Pear</li>
</ul>
This is the HTML markup we will be using in all of the API examples.
Loading
First you need to load in the HTML. This step in jQuery is implicit, since jQuery operates on the one, baked-in DOM. With Cheerio, we need to pass in the HTML document.
This is the preferred method:
// ES6 or TypeScript:
import * as cheerio from 'cheerio';
// In other environments:
const cheerio = require('cheerio');
const $ = cheerio.load('<ul id="fruits">...</ul>');
$.html();
//=> <html><head></head><body><ul id="fruits">...</ul></body></html>
Similar to web browser contexts, load
will introduce <html>
, <head>
, and <body>
elements if they are not already present. You can set load
's third argument to false
to disable this.
const $ = cheerio.load('<ul id="fruits">...</ul>', null, false);
$.html();
//=> '<ul id="fruits">...</ul>'
Optionally, you can also load in the HTML by passing the string as the context:
$('ul', '<ul id="fruits">...</ul>');
Or as the root:
$('li', 'ul', '<ul id="fruits">...</ul>');
If you need to modify parsing options for XML input, you may pass an extra
object to .load()
:
const $ = cheerio.load('<ul id="fruits">...</ul>', {
xml: {
normalizeWhitespace: true,
},
});
The options in the xml
object are taken directly from htmlparser2, therefore any options that can be used in htmlparser2
are valid in cheerio as well. When xml
is set, the default options are:
{
xmlMode: true,
decodeEntities: true, // Decode HTML entities.
withStartIndices: false, // Add a `startIndex` property to nodes.
withEndIndices: false, // Add an `endIndex` property to nodes.
}
For a full list of options and their effects, see domhandler and htmlparser2's options.
Using htmlparser2
Cheerio ships with two parsers, parse5
and htmlparser2
. The
former is the default for HTML, the latter the default for XML.
Some users may wish to parse markup with the htmlparser2
library, and
traverse/manipulate the resulting structure with Cheerio. This may be the case
for those upgrading from pre-1.0 releases of Cheerio (which relied on
htmlparser2
), for those dealing with invalid markup (because htmlparser2
is
more forgiving), or for those operating in performance-critical situations
(because htmlparser2
may be faster in some cases). Note that "more forgiving"
means htmlparser2
has error-correcting mechanisms that aren't always a match
for the standards observed by web browsers. This behavior may be useful when
parsing non-HTML content.
To support these cases, load
also accepts a htmlparser2
-compatible data
structure as its first argument. Users may install htmlparser2
, use it to
parse input, and pass the result to load
:
// Usage as of htmlparser2 version 6:
const htmlparser2 = require('htmlparser2');
const dom = htmlparser2.parseDocument(document, options);
const $ = cheerio.load(dom);
If you want to save some bytes, you can use Cheerio's slim export, which
always uses htmlparser2
:
const cheerio = require('cheerio/lib/slim');
Selectors
Cheerio's selector implementation is nearly identical to jQuery's, so the API is very similar.
\$( selector, [context], [root] )
selector
searches within the context
scope which searches within the root
scope. selector
and context
can be a string expression, DOM Element, array of DOM elements, or cheerio object. root
is typically the HTML document string.
This selector method is the starting point for traversing and manipulating the document. Like jQuery, it's the primary method for selecting elements in the document.
$('.apple', '#fruits').text();
//=> Apple
$('ul .pear').attr('class');
//=> pear
$('li[class=orange]').html();
//=> Orange
XML Namespaces
You can select with XML Namespaces but due to the CSS specification, the colon (:
) needs to be escaped for the selector to be valid.
$('[xml\\:id="main"');
Rendering
When you're ready to render the document, you can call the html
method on the "root" selection:
$.root().html();
//=> <html>
// <head></head>
// <body>
// <ul id="fruits">
// <li class="apple">Apple</li>
// <li class="orange">Orange</li>
// <li class="pear">Pear</li>
// </ul>
// </body>
// </html>
If you want to render the outerHTML
of a selection, you can use the html
utility functon:
cheerio.html($('.pear'));
//=> <li class="pear">Pear</li>
You may also render the text content of a Cheerio object using the text
static method:
const $ = cheerio.load('This is <em>content</em>.');
cheerio.text($('body'));
//=> This is content.
Plugins
Once you have loaded a document, you may extend the prototype or the equivalent fn
property with custom plugin methods:
const $ = cheerio.load('<html><body>Hello, <b>world</b>!</body></html>');
$.prototype.logHtml = function () {
console.log(this.html());
};
$('body').logHtml(); // logs "Hello, <b>world</b>!" to the console
If you're using TypeScript, you should add a type definition for your new method:
declare module 'cheerio' {
interface Cheerio<T> {
logHtml(this: Cheerio<T>): void;
}
}
The "DOM Node" object
Cheerio collections are made up of objects that bear some resemblance to browser-based DOM nodes. You can expect them to define the following properties:
tagName
parentNode
previousSibling
nextSibling
nodeValue
firstChild
childNodes
lastChild
Screencasts
This video tutorial is a follow-up to Nettut's "How to Scrape Web Pages with Node.js and jQuery", using cheerio instead of JSDOM + jQuery. This video shows how easy it is to use cheerio and how much faster cheerio is than JSDOM + jQuery.
Cheerio in the real world
Are you using cheerio in production? Add it to the wiki!
Sponsors
Does your company use Cheerio in production? Please consider sponsoring this project! Your help will allow maintainers to dedicate more time and resources to its development and support.
<!-- BEGIN SPONSORS: sponsor -->
<!-- END SPONSORS -->
Backers
Become a backer to show your support for Cheerio and help us maintain and improve this open source project.
<!-- BEGIN SPONSORS: backer -->
<!-- END SPONSORS -->
Special Thanks
This library stands on the shoulders of some incredible developers. A special thanks to:
• @FB55 for node-htmlparser2 & CSSSelect:
Felix has a knack for writing speedy parsing engines. He completely re-wrote both @tautologistic's node-htmlparser
and @harry's node-soupselect
from the ground up, making both of them much faster and more flexible. Cheerio would not be possible without his foundational work
• @jQuery team for jQuery: The core API is the best of its class and despite dealing with all the browser inconsistencies the code base is extremely clean and easy to follow. Much of cheerio's implementation and documentation is from jQuery. Thanks guys.
• @visionmedia: The style, the structure, the open-source"-ness" of this library comes from studying TJ's style and using many of his libraries. This dude consistently pumps out high-quality libraries and has always been more than willing to help or answer questions. You rock TJ.
License
MIT
*Note that all licence references and agreements mentioned in the cheerio README section above
are relevant to that project's source code only.