execa alternatives and similar modules
Based on the "Miscellaneous" category.
Alternatively, view execa alternatives based on common mentions on social networks and blogs.
-
mem
Memoize functions - an optimization technique used to speed up consecutive function calls by caching the result of calls with identical input -
basic-ftp
FTP client for Node.js, supports FTPS over TLS, passive mode over IPv6, async/await, and Typescript. -
schemapack
Create a schema object to encode/decode your JSON in to a compact byte buffer with no overhead. -
nar
node.js application archive - create self-contained binary like executable applications that are ready to ship and run -
Faster than fast, smaller than micro ... nano-memoizer.
Faster than fast, smaller than micro ... a nano speed and size (780 Brotili bytes) memoize for single and multiple argument functions.
SurveyJS - Open-Source JSON Form Builder to Create Dynamic Forms Right in Your App
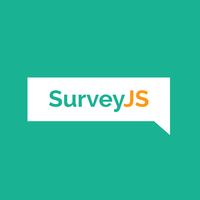
* Code Quality Rankings and insights are calculated and provided by Lumnify.
They vary from L1 to L5 with "L5" being the highest.
Do you think we are missing an alternative of execa or a related project?
Popular Comparisons
README
Process execution for humans
Why
This package improves child_process
methods with:
- Promise interface.
- Strips the final newline from the output so you don't have to do
stdout.trim()
. - Supports shebang binaries cross-platform.
- Improved Windows support.
- Higher max buffer. 100 MB instead of 200 KB.
- Executes locally installed binaries by name.
- Cleans up spawned processes when the parent process dies.
- Get interleaved output from
stdout
andstderr
similar to what is printed on the terminal. (Async only) - Can specify file and arguments as a single string without a shell
- More descriptive errors.
Install
npm install execa
Usage
import {execa} from 'execa';
const {stdout} = await execa('echo', ['unicorns']);
console.log(stdout);
//=> 'unicorns'
Pipe the child process stdout to the parent
import {execa} from 'execa';
execa('echo', ['unicorns']).stdout.pipe(process.stdout);
Handling Errors
import {execa} from 'execa';
// Catching an error
try {
await execa('unknown', ['command']);
} catch (error) {
console.log(error);
/*
{
message: 'Command failed with ENOENT: unknown command spawn unknown ENOENT',
errno: -2,
code: 'ENOENT',
syscall: 'spawn unknown',
path: 'unknown',
spawnargs: ['command'],
originalMessage: 'spawn unknown ENOENT',
shortMessage: 'Command failed with ENOENT: unknown command spawn unknown ENOENT',
command: 'unknown command',
escapedCommand: 'unknown command',
stdout: '',
stderr: '',
all: '',
failed: true,
timedOut: false,
isCanceled: false,
killed: false
}
*/
}
Cancelling a spawned process
import {execa} from 'execa';
const abortController = new AbortController();
const subprocess = execa('node', [], {signal: abortController.signal});
setTimeout(() => {
abortController.abort();
}, 1000);
try {
await subprocess;
} catch (error) {
console.log(subprocess.killed); // true
console.log(error.isCanceled); // true
}
Catching an error with the sync method
import {execaSync} from 'execa';
try {
execaSync('unknown', ['command']);
} catch (error) {
console.log(error);
/*
{
message: 'Command failed with ENOENT: unknown command spawnSync unknown ENOENT',
errno: -2,
code: 'ENOENT',
syscall: 'spawnSync unknown',
path: 'unknown',
spawnargs: ['command'],
originalMessage: 'spawnSync unknown ENOENT',
shortMessage: 'Command failed with ENOENT: unknown command spawnSync unknown ENOENT',
command: 'unknown command',
escapedCommand: 'unknown command',
stdout: '',
stderr: '',
all: '',
failed: true,
timedOut: false,
isCanceled: false,
killed: false
}
*/
}
Kill a process
Using SIGTERM, and after 2 seconds, kill it with SIGKILL.
const subprocess = execa('node');
setTimeout(() => {
subprocess.kill('SIGTERM', {
forceKillAfterTimeout: 2000
});
}, 1000);
API
execa(file, arguments, options?)
Execute a file. Think of this as a mix of child_process.execFile()
and child_process.spawn()
.
No escaping/quoting is needed.
Unless the shell
option is used, no shell interpreter (Bash, cmd.exe
, etc.) is used, so shell features such as variables substitution (echo $PATH
) are not allowed.
Returns a child_process
instance which:
- is also a
Promise
resolving or rejecting with achildProcessResult
. - exposes the following additional methods and properties.
kill(signal?, options?)
Same as the original child_process#kill()
except: if signal
is SIGTERM
(the default value) and the child process is not terminated after 5 seconds, force it by sending SIGKILL
.
options.forceKillAfterTimeout
Type: number | false
\
Default: 5000
Milliseconds to wait for the child process to terminate before sending SIGKILL
.
Can be disabled with false
.
all
Type: ReadableStream | undefined
Stream combining/interleaving stdout
and stderr
.
This is undefined
if either:
- the
all
option isfalse
(the default value) - both
stdout
andstderr
options are set to'inherit'
,'ipc'
,Stream
orinteger
execaSync(file, arguments?, options?)
Execute a file synchronously.
Returns or throws a childProcessResult
.
execaCommand(command, options?)
Same as execa()
except both file and arguments are specified in a single command
string. For example, execa('echo', ['unicorns'])
is the same as execaCommand('echo unicorns')
.
If the file or an argument contains spaces, they must be escaped with backslashes. This matters especially if command
is not a constant but a variable, for example with __dirname
or process.cwd()
. Except for spaces, no escaping/quoting is needed.
The shell
option must be used if the command
uses shell-specific features (for example, &&
or ||
), as opposed to being a simple file
followed by its arguments
.
execaCommandSync(command, options?)
Same as execaCommand()
but synchronous.
Returns or throws a childProcessResult
.
execaNode(scriptPath, arguments?, options?)
Execute a Node.js script as a child process.
Same as execa('node', [scriptPath, ...arguments], options)
except (like child_process#fork()
):
- the current Node version and options are used. This can be overridden using the
nodePath
andnodeOptions
options. - the
shell
option cannot be used - an extra channel
ipc
is passed tostdio
childProcessResult
Type: object
Result of a child process execution. On success this is a plain object. On failure this is also an Error
instance.
The child process fails when:
- its exit code is not
0
- it was killed with a signal
- timing out
- being canceled
- there's not enough memory or there are already too many child processes
command
Type: string
The file and arguments that were run, for logging purposes.
This is not escaped and should not be executed directly as a process, including using execa()
or execaCommand()
.
escapedCommand
Type: string
Same as command
but escaped.
This is meant to be copy and pasted into a shell, for debugging purposes.
Since the escaping is fairly basic, this should not be executed directly as a process, including using execa()
or execaCommand()
.
exitCode
Type: number
The numeric exit code of the process that was run.
stdout
Type: string | Buffer
The output of the process on stdout.
stderr
Type: string | Buffer
The output of the process on stderr.
all
Type: string | Buffer | undefined
The output of the process with stdout
and stderr
interleaved.
This is undefined
if either:
- the
all
option isfalse
(the default value) execaSync()
was used
failed
Type: boolean
Whether the process failed to run.
timedOut
Type: boolean
Whether the process timed out.
isCanceled
Type: boolean
Whether the process was canceled.
You can cancel the spawned process using the signal
option.
killed
Type: boolean
Whether the process was killed.
signal
Type: string | undefined
The name of the signal that was used to terminate the process. For example, SIGFPE
.
If a signal terminated the process, this property is defined and included in the error message. Otherwise it is undefined
.
signalDescription
Type: string | undefined
A human-friendly description of the signal that was used to terminate the process. For example, Floating point arithmetic error
.
If a signal terminated the process, this property is defined and included in the error message. Otherwise it is undefined
. It is also undefined
when the signal is very uncommon which should seldomly happen.
message
Type: string
Error message when the child process failed to run. In addition to the underlying error message, it also contains some information related to why the child process errored.
The child process stderr then stdout are appended to the end, separated with newlines and not interleaved.
shortMessage
Type: string
This is the same as the message
property except it does not include the child process stdout/stderr.
originalMessage
Type: string | undefined
Original error message. This is the same as the message
property except it includes neither the child process stdout/stderr nor some additional information added by Execa.
This is undefined
unless the child process exited due to an error
event or a timeout.
options
Type: object
cleanup
Type: boolean
\
Default: true
Kill the spawned process when the parent process exits unless either:
- the spawned process is detached
- the parent process is terminated abruptly, for example, with SIGKILL
as opposed to SIGTERM
or a normal exit
preferLocal
Type: boolean
\
Default: false
Prefer locally installed binaries when looking for a binary to execute.\
If you $ npm install foo
, you can then execa('foo')
.
localDir
Type: string | URL
\
Default: process.cwd()
Preferred path to find locally installed binaries in (use with preferLocal
).
execPath
Type: string
\
Default: process.execPath
(Current Node.js executable)
Path to the Node.js executable to use in child processes.
This can be either an absolute path or a path relative to the cwd
option.
Requires preferLocal
to be true
.
For example, this can be used together with get-node
to run a specific Node.js version in a child process.
buffer
Type: boolean
\
Default: true
Buffer the output from the spawned process. When set to false
, you must read the output of stdout
and stderr
(or all
if the all
option is true
). Otherwise the returned promise will not be resolved/rejected.
If the spawned process fails, error.stdout
, error.stderr
, and error.all
will contain the buffered data.
input
Type: string | Buffer | stream.Readable
Write some input to the stdin
of your binary.\
Streams are not allowed when using the synchronous methods.
stdin
Type: string | number | Stream | undefined
\
Default: pipe
Same options as stdio
.
stdout
Type: string | number | Stream | undefined
\
Default: pipe
Same options as stdio
.
stderr
Type: string | number | Stream | undefined
\
Default: pipe
Same options as stdio
.
all
Type: boolean
\
Default: false
Add an .all
property on the promise and the resolved value. The property contains the output of the process with stdout
and stderr
interleaved.
reject
Type: boolean
\
Default: true
Setting this to false
resolves the promise with the error instead of rejecting it.
stripFinalNewline
Type: boolean
\
Default: true
Strip the final newline character from the output.
extendEnv
Type: boolean
\
Default: true
Set to false
if you don't want to extend the environment variables when providing the env
property.
Execa also accepts the below options which are the same as the options for child_process#spawn()
/child_process#exec()
cwd
Type: string | URL
\
Default: process.cwd()
Current working directory of the child process.
env
Type: object
\
Default: process.env
Environment key-value pairs. Extends automatically from process.env
. Set extendEnv
to false
if you don't want this.
argv0
Type: string
Explicitly set the value of argv[0]
sent to the child process. This will be set to file
if not specified.
stdio
Type: string | string[]
\
Default: pipe
Child's stdio configuration.
serialization
Type: string
\
Default: 'json'
Specify the kind of serialization used for sending messages between processes when using the stdio: 'ipc'
option or execaNode()
:
- json
: Uses JSON.stringify()
and JSON.parse()
.
- advanced
: Uses v8.serialize()
detached
Type: boolean
Prepare child to run independently of its parent process. Specific behavior depends on the platform.
uid
Type: number
Sets the user identity of the process.
gid
Type: number
Sets the group identity of the process.
shell
Type: boolean | string
\
Default: false
If true
, runs file
inside of a shell. Uses /bin/sh
on UNIX and cmd.exe
on Windows. A different shell can be specified as a string. The shell should understand the -c
switch on UNIX or /d /s /c
on Windows.
We recommend against using this option since it is:
- not cross-platform, encouraging shell-specific syntax.
- slower, because of the additional shell interpretation.
- unsafe, potentially allowing command injection.
encoding
Type: string | null
\
Default: utf8
Specify the character encoding used to decode the stdout
and stderr
output. If set to null
, then stdout
and stderr
will be a Buffer
instead of a string.
timeout
Type: number
\
Default: 0
If timeout is greater than 0
, the parent will send the signal identified by the killSignal
property (the default is SIGTERM
) if the child runs longer than timeout milliseconds.
maxBuffer
Type: number
\
Default: 100_000_000
(100 MB)
Largest amount of data in bytes allowed on stdout
or stderr
.
killSignal
Type: string | number
\
Default: SIGTERM
Signal value to be used when the spawned process will be killed.
signal
Type: AbortSignal
You can abort the spawned process using AbortController
.
When AbortController.abort()
is called, .isCanceled
becomes false
.
Requires Node.js 16 or later.
windowsVerbatimArguments
Type: boolean
\
Default: false
If true
, no quoting or escaping of arguments is done on Windows. Ignored on other platforms. This is set to true
automatically when the shell
option is true
.
windowsHide
Type: boolean
\
Default: true
On Windows, do not create a new console window. Please note this also prevents CTRL-C
from working on Windows.
nodePath (For .node()
only)
Type: string
\
Default: process.execPath
Node.js executable used to create the child process.
nodeOptions (For .node()
only)
Type: string[]
\
Default: process.execArgv
List of CLI options passed to the Node.js executable.
Tips
Retry on error
Gracefully handle failures by using automatic retries and exponential backoff with the p-retry
package:
import pRetry from 'p-retry';
const run = async () => {
const results = await execa('curl', ['-sSL', 'https://sindresorhus.com/unicorn']);
return results;
};
console.log(await pRetry(run, {retries: 5}));
Save and pipe output from a child process
Let's say you want to show the output of a child process in real-time while also saving it to a variable.
import {execa} from 'execa';
const subprocess = execa('echo', ['foo']);
subprocess.stdout.pipe(process.stdout);
const {stdout} = await subprocess;
console.log('child output:', stdout);
Redirect output to a file
import {execa} from 'execa';
const subprocess = execa('echo', ['foo'])
subprocess.stdout.pipe(fs.createWriteStream('stdout.txt'))
Redirect input from a file
import {execa} from 'execa';
const subprocess = execa('cat')
fs.createReadStream('stdin.txt').pipe(subprocess.stdin)
Execute the current package's binary
import {getBinPathSync} from 'get-bin-path';
const binPath = getBinPathSync();
const subprocess = execa(binPath);
execa
can be combined with get-bin-path
to test the current package's binary. As opposed to hard-coding the path to the binary, this validates that the package.json
bin
field is correctly set up.
Related
- gulp-execa - Gulp plugin for
execa
- nvexeca - Run
execa
using any Node.js version - sudo-prompt - Run commands with elevated privileges.
Maintainers
Get professional support for this package with a Tidelift subscription Tidelift helps make open source sustainable for maintainers while giving companiesassurances about security, maintenance, and licensing for their dependencies.